November 18, 2010, 17:03
Hello
here is one other way to write a kios mode .NET application using a technique called SubClassing. The idea was born by a comment of redwolf2222 on this blog about how to Hide Start and Close buttons on Windows Mobile 6.5 devices. Redwolf2222 also provided a code snippet. Unfortunately it was incomplete and so I wrote my own class.
Disable clicks on Start and Close button
The demo project shows one dialog with two check boxes and you can easily test the function. If “StartButton Disabled” or “Close Button disabled” is checked, you cannot ‘click’ the corresponding button any more:
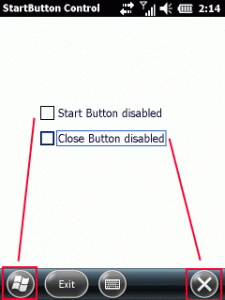
You still ‘click’ the buttons but the subclassed window will not ‘execute’ your click. The buttons are part of the toolbar32 window which is a child of the menu_worker window. So first we have to follow the window tree.
Find the right window
/// <summary>
/// SubClassing: Install the wndproc hook
/// </summary>
/// <returns></returns>
private bool hookWindow()
{
//find taskbar
IntPtr hWndTaskbar = FindWindow("HHTaskbar", IntPtr.Zero);
if (hWndTaskbar == IntPtr.Zero)
return false;
//enable the taskbar, not realy necessary
EnableWindow(hWndTaskbar, true);
//already installed?
if (oldWndProc == IntPtr.Zero)
{
//find the menu_worker window
IntPtr hwndMenu_Worker = FindWindow("menu_worker", IntPtr.Zero);
if (hwndMenu_Worker != IntPtr.Zero)
{
//get the child window which has the buttons on it
IntPtr hwndToolbar = GetWindow(hwndMenu_Worker, GetWindow_Cmd.GW_CHILD);
if (hwndToolbar != IntPtr.Zero)
{
_mHwnd = hwndToolbar; //store to remember
SubclassHWnd(hwndToolbar); //subclass the wndproc
}
}
}
return true;
}
Subclassing
Now, as we have the window handle, the subclassing can be started:
Continue reading ‘Mobile Development: Disable Windows Mobile 6.5 Start and Close Button’ »
Tags:
6.5.3,
Close Button,
DotNet,
hook,
kiosk mode,
Programming,
sender-as-rectangle-windows-ce,
Start Button,
Subclassing,
taskbar,
windows mobile,
WM65 Category:
CodeProject,
kiosk mode,
Programming,
Tools,
Utilities |
Comments Off on Mobile Development: Disable Windows Mobile 6.5 Start and Close Button
July 17, 2010, 08:15
Hi there
I would like to bring back to mind the advantages of using a layout manager (LM) for your smart device .Net projects.
None of the layout Managers mentioned here are developed by me, I dont have the time to do so, especially, when there are great free solutions on the net.
On full .net framework you have the tablelayout panel where you can place your GUI elements and the elements will be arranged automatically, when the main form is resized.
OK, on smart devices, there will be less form resizing as mostly the forms are full screen by design (QVGA:240×320 or VGA:480×640) . But what happens to your form design, when the user rotates the screen from portrait to landscape orientation?
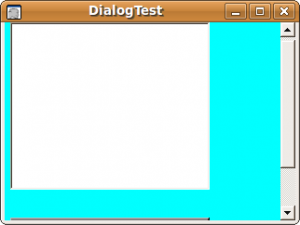
Continue reading ‘Mobile Development-Using Layout Managers’ »
Tags:
.Net,
coding,
DotNet,
GUI,
Landscape,
Layout Manager,
Portrait,
QVGA,
Smart Device,
VGA Category:
CodeProject,
Programming,
Tips |
Comments Off on Mobile Development-Using Layout Managers
June 1, 2010, 19:55
Although there are well know ways to update the GUI from a background thread not running in the GUI thread, I looked for an easiest to use solution. After some experiments I got a background thread class that is very easy to use. No BeginInvoke or Control.Invoke needed any more to update a GUI element.
The final solution uses a MessageWindow inside a control based class with a worker thread. As the control and the MessageWindow is part of the GUI thread, there is no need to use Invokes.
Inside the thread I use SendMessage to transfer background thread informations to the control, which then fires an event you can subscribe in the GUI thread.
The test app attached shows three threads running independently and all update the GUI frequently without blocking the GUI.
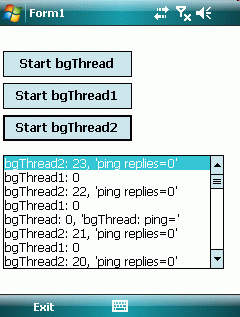
Here is my try to visualize my idea and solution
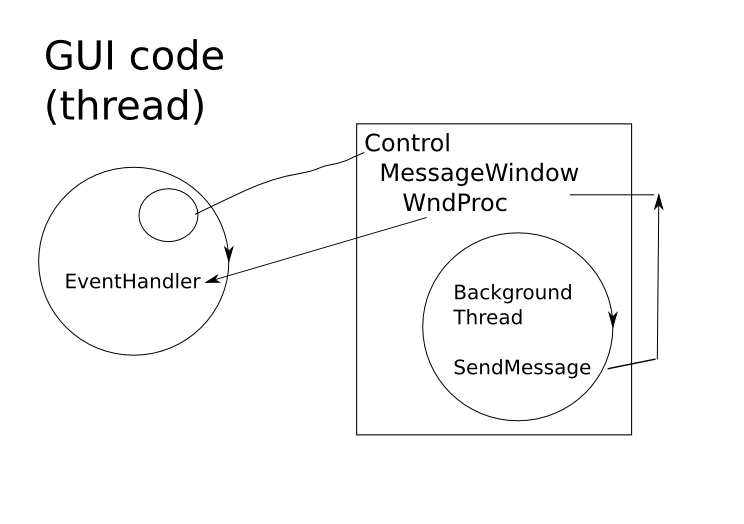
Continue reading ‘Mobile Development: Easy to use background thread with GUI update’ »
Tags:
Background Thread,
C#,
Compact Framework,
DotNet,
event handler,
GUI thread,
Invoke,
MessageWindow,
Mobile Development,
SendMessage,
WM_COPYDATA,
WndProc,
Worker Thread Category:
CodeProject,
Programming |
Comments Off on Mobile Development: Easy to use background thread with GUI update